源码已经打包在博客末尾,有需要的话自行下载。
java 项目: 客户信息管理系统
环境:
Idea 集成开发工具
技术点:
- 面向对象
- 数组
项目结构:
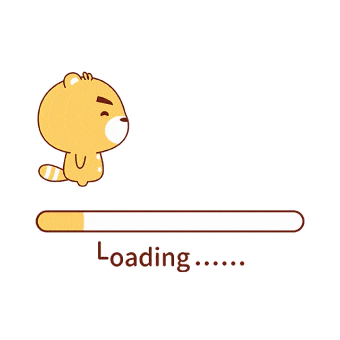
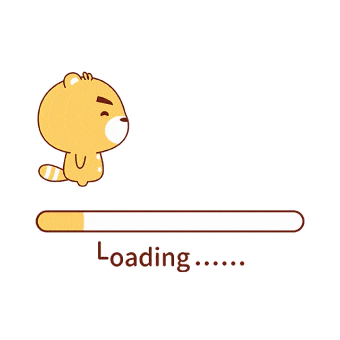
代码展示:
【bean】 Customer.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| public class Customer { private String name; private char gender; private int age; private String phone; private String email;
public Customer() { }
public Customer(String name, char gender, int age, String phone, String email) { this.name = name; this.gender = gender; this.age = age; this.phone = phone; this.email = email; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public char getGender() { return gender; }
public void setGender(char gender) { this.gender = gender; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public String getPhone() { return phone; }
public void setPhone(String phone) { this.phone = phone; }
public String getEmail() { return email; }
public void setEmail(String email) { this.email = email; } }
|
【service】 CusomerList.java\
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94
|
public class CustomerList { private Customer[] customers; private int total;
public CustomerList(int totalCustomer){ customers = new Customer[totalCustomer]; }
public boolean addCustomer(Customer customer){ if(customer != null && total < customers.length){ customers[total++] = customer; return true; } return false; }
public boolean replanceCustomer(int index, Customer cust){ if(index >= 0 && index < total){ customers[index] = cust; return true; } return false; }
public boolean deleteCustomer(int index){ if(index >= 0 && index < total){ for(int i = index; i < total -1; i++){ customers[i] = customers[i+1]; } customers[total -1] = null; total--; return true; } return false; }
public Customer[] getAllCustomers(){ Customer[] custs = new Customer[total]; for(int i = 0; i < custs.length; i++){ custs[i] = customers[i]; } return custs; }
public Customer getCustomer(int index){ if(index >= 0 && index < total){ return customers[index]; } return null; }
public int getToTal(){ return total; } }
|
【ul】 CustomerView.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179
| public class CustomerView { private CustomerList customerList = new CustomerList(10);
public CustomerView(){ Customer customer = new Customer("柯南",'男',23,"13344453434","kennan@123.com"); customerList.addCustomer(customer); }
public void enterMainMenu(){ boolean isFlag = true; do{ System.out.println("\n-----------------客户信息管理软件-----------------\n"); System.out.println(" 1 添 加 客 户"); System.out.println(" 2 修 改 客 户"); System.out.println(" 3 删 除 客 户"); System.out.println(" 4 客 户 列 表"); System.out.println(" 5 退 出\n"); System.out.print(" 请选择(1-5):"); char menu = CMUtility.readMenuSelection(); switch(menu){ case '1': addNewCustomer(); break; case '2': modifyCustomer(); break; case '3': deleteCustomer(); break; case '4': listAllCustomer(); break; case '5': System.out.print("确认是否退出(Y/N):"); char exit = CMUtility.readConfirmSelection(); if(exit == 'Y'){ isFlag = false; } } }while(isFlag); }
public void addNewCustomer(){ System.out.println("---------------------添加客户---------------------"); System.out.print("姓名:"); String name = CMUtility.readString(5); System.out.print("性别:"); char gender = CMUtility.readChar(); System.out.print("年龄:"); int age = CMUtility.readInt(); System.out.print("电话:"); String phone = CMUtility.readString(13); System.out.print("邮箱:"); String email = CMUtility.readString(20);
Customer customer = new Customer(name, gender, age, phone, email); boolean flag = customerList.addCustomer(customer); if(flag){ System.out.println("---------------------添加完成---------------------"); }else{ System.out.println("人数已达上限,添加失败!"); } }
public void modifyCustomer(){ System.out.println("---------------------修改客户---------------------"); Customer customer; int index; for(;;){ System.out.print("请选择待修改客户编号(-1退出):"); index = CMUtility.readInt(); if(index == -1){ return; } customer = customerList.getCustomer(index -1); if(customer == null){ System.out.println("无法找到指定客户!"); }else{ break; } } System.out.println("姓名(" + customer.getName() + "):"); String name = CMUtility.readString(5, customer.getName()); System.out.println("性别(" + customer.getGender() + "):"); char gender = CMUtility.readChar(customer.getGender()); System.out.println("年龄(" + customer.getAge() + "):"); int age = CMUtility.readInt(customer.getAge()); System.out.println("电话(" + customer.getPhone() + "):"); String phone = CMUtility.readString(13, customer.getPhone()); System.out.println("邮箱(" + customer.getEmail() + "):"); String email = CMUtility.readString(20, customer.getEmail());
customer = new Customer(name, gender, age, phone, email);
boolean flag = customerList.replanceCustomer(index -1, customer); if(flag){ System.out.println("---------------------修改完成---------------------"); }else{ System.out.println("---------------------修改失败---------------------"); } }
public void deleteCustomer(){ System.out.println("---------------------删除客户---------------------"); Customer customer; int index; for(;;){ System.out.println("请选择待删除客户编号(-1退出):"); index = CMUtility.readInt(); if(index == -1){ return; } customer = customerList.getCustomer(index -1); if(customer == null){ System.out.println("无法找到指定的客户!"); }else{ break; } }
System.out.println("确认是否删除(Y/N):"); char deleOrNot = CMUtility.readChar(); if(deleOrNot == 'Y'){ boolean flag = customerList.deleteCustomer(index -1); if(flag){ System.out.println("---------------------删除完成---------------------"); }else{ System.out.println("---------------------删除失败---------------------"); } }else{ return; } }
public void listAllCustomer(){ System.out.println("---------------------------客户列表---------------------------"); Customer[] customers = customerList.getAllCustomers(); if(customers.length == 0){ System.out.println("没有任何客户记录!"); }else{ System.out.println("编号\t\t姓名\t\t性别\t\t年龄\t\t电话\t\t\t\t邮箱"); for(int i = 0; i < customers.length; i++){ Customer cust = customers[i]; System.out.println((i + 1) + "\t\t" + cust.getName() + "\t\t" + cust.getGender() + "\t\t" + cust.getAge() + "\t\t" + cust.getPhone() + "\t\t" + cust.getEmail()); } } System.out.println("-------------------------客户列表完成-------------------------"); }
public static void main(String[] args){ CustomerView view = new CustomerView(); view.enterMainMenu(); } }
|
【util】 CMUtility.java 工具类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126
| import java.util.*;
public class CMUtility { private static Scanner scanner = new Scanner(System.in);
public static char readMenuSelection() { char c; for (; ; ) { String str = readKeyBoard(1, false); c = str.charAt(0); if (c != '1' && c != '2' && c != '3' && c != '4' && c != '5') { System.out.print("选择错误,请重新输入:"); } else break; } return c; }
public static char readChar() { String str = readKeyBoard(1, false); return str.charAt(0); }
public static char readChar(char defaultValue) { String str = readKeyBoard(1, true); return (str.length() == 0) ? defaultValue : str.charAt(0); }
public static int readInt() { int n; for (; ; ) { String str = readKeyBoard(2, false); try { n = Integer.parseInt(str); break; } catch (NumberFormatException e) { System.out.print("数字输入错误,请重新输入:"); } } return n; }
public static int readInt(int defaultValue) { int n; for (; ; ) { String str = readKeyBoard(2, true); if (str.equals("")) { return defaultValue; }
try { n = Integer.parseInt(str); break; } catch (NumberFormatException e) { System.out.print("数字输入错误,请重新输入:"); } } return n; }
public static String readString(int limit) { return readKeyBoard(limit, false); }
public static String readString(int limit, String defaultValue) { String str = readKeyBoard(limit, true); return str.equals("")? defaultValue : str; }
public static char readConfirmSelection() { char c; for (; ; ) { String str = readKeyBoard(1, false).toUpperCase(); c = str.charAt(0); if (c == 'Y' || c == 'N') { break; } else { System.out.print("选择错误,请重新输入:"); } } return c; }
private static String readKeyBoard(int limit, boolean blankReturn) { String line = "";
while (scanner.hasNextLine()) { line = scanner.nextLine(); if (line.length() == 0) { if (blankReturn) return line; else continue; }
if (line.length() < 1 || line.length() > limit) { System.out.print("输入长度(不大于" + limit + ")错误,请重新输入:"); continue; } break; }
return line; } }
|
提取码:qrj3