语雀文档库: https://www.yuque.com/imoyt/zssuuf
一.、Vue
安装 vue
1 2
| # 最新稳定版 $ npm install vue
|
1、vue 声明式渲染
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| let vm = new Vue({ el: "#app", data: { name: "张三", num: 1, }, methods: { cancle() { this.num--; }, hello() { return "1"; }, }, });
|
2、双向绑定,模型变化,视图变化。反之亦然
双向绑定使用 v-model
1
| <input type="text" v-model="num" />
|
1
| <h1> {{name}} ,非常帅,有{{num}}个人为他点赞{{hello()}}</h1>
|
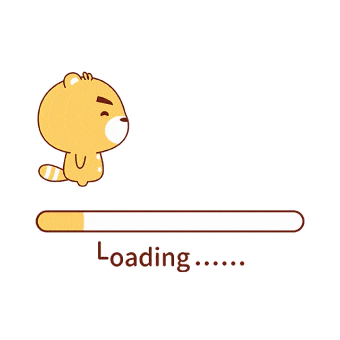
3、事件处理
v-xx:指令
1、创建 vue 实例,关联页面的模板,将自己的数据(data)渲染到关联的模板,响应式的
2、指令来简化对 dom 的一些操作。
3、声明方法来做更复杂的操作。methods 里面可以封装方法。
v-on 是按钮的单击事件:
1
| <button v-on:click="num++">点赞</button>
|
在 VUE 中 el,data 和 vue 的作用:
- el:用来绑定数据;
- data:用来封装数据;
- methods:用来封装方法,并且能够封装多个方法,如何上面封装了 cancell 和 hello 方法。
安装“Vue 2 Snippets”,用来做代码提示
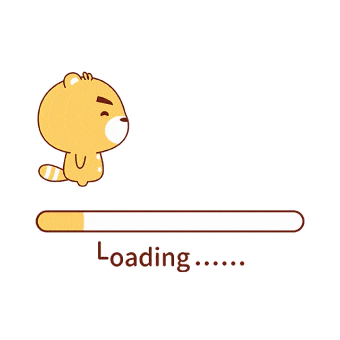
为了方便的在浏览器上调试 VUE 程序,需要安装“vue-devtools”,编译后安装到 chrome 中即可。
详细的使用方法见:Vue 调试神器 vue-devtools 安装
“v-html”不会对于 HTML 标签进行转义,而是直接在浏览器上显示 data 所设置的内容;而“ v-text”会对 html 标签进行转义
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <div id="app"> {{msg}} {{1+1}} {{hello()}}<br/> <span v-html="msg"></span> <br/> <span v-text="msg"></span> </div>
<script src="../node_modules/vue/dist/vue.js"></script>
<script> new Vue({ el:"#app", data:{ msg:"<h1>Hello</h1>", link:"http://www.baidu.com" }, methods:{ hello(){ return "World" } } }) </script>
|
运行结果:
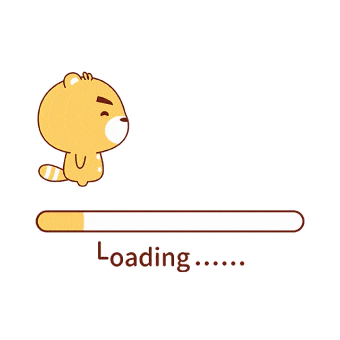
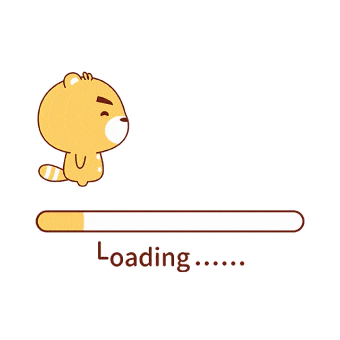
4、v-bind :单向绑定
给 html 标签的属性绑定
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <!-- 给html标签的属性绑定 --> <div id="app">
<a v-bind:href="link">gogogo</a>
<span v-bind:class="{active:isActive,'text-danger':hasError}" :style="{color: color1,fontSize: size}">你好</span>
</div>
<script src="../node_modules/vue/dist/vue.js"></script>
<script> let vm = new Vue({ el:"#app", data:{ link: "http://www.baidu.com", isActive:true, hasError:true, color1:'red', size:'36px' } }) </script>
|
上面所完成的任务就是给 a 标签绑定一个超链接。并且当“isActive”和“hasError”都是 true 的时候,将属性动态的绑定到,则绑定该“active”和 “text-danger”class。这样可以动态的调整属性的存在。
而且如果想要实现修改 vm 的”color1”和“size”, span 元素的 style 也能够随之变化,则可以写作 v-bind:style,也可以省略 v-bind。
5、v-model 双向绑定
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head> <body> <div id="app"> 精通的语言: <input type="checkbox" v-model="language" value="Java" /> java<br /> <input type="checkbox" v-model="language" value="PHP" /> PHP<br /> <input type="checkbox" v-model="language" value="Python" /> Python<br /> 选中了 \{{language.join(",")}} </div>
<script src="../node_modules/vue/dist/vue.js"></script>
<script> let vm = new Vue({ el: "#app", data: { language: [], }, }); </script> </body> </html>
|
上面完成的功能就是通过“v-model”为输入框绑定多个值,能够实现选中的值,在 data 的 language 也在不断的发生着变化,
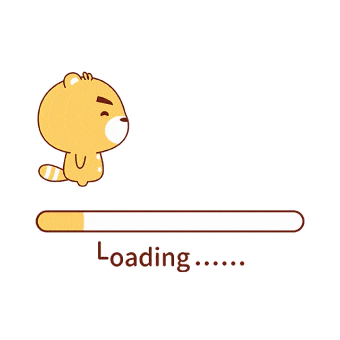
如果在控制台上指定 vm.language=[“Java”,”PHP”],则 data 值也会跟着变化。
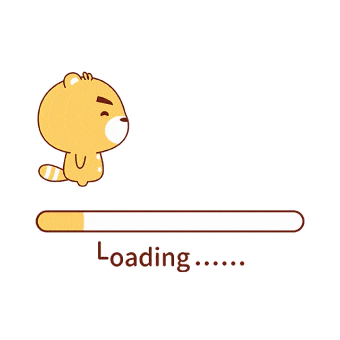
通过“v-model”实现了页面发生了变化,则数据也发生变化,数据发生变化,则页面也发生变化,这样就实现了双向绑定。
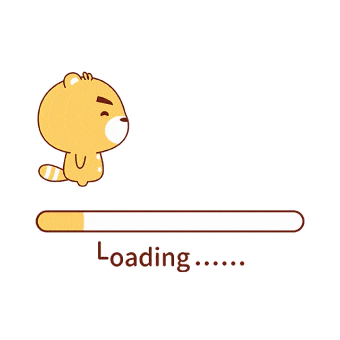
6、v-on 为按钮绑定事件
1 2 3 4
| <!--事件中直接写js片段--> <button v-on:click="num++">点赞</button> <!--事件指定一个回调函数,必须是Vue实例中定义的函数--> <button @click="cancle">取消</button>
|
上面是为两个按钮绑定了单击事件,其中一个对于 num 进行自增,另外一个自减。
v-on:click 也可以写作@click
事件的冒泡:
1 2 3 4 5 6 7 8
| <div style="border: 1px solid red;padding: 20px;" v-on:click="hello"> 大div <div style="border: 1px solid blue;padding: 20px;" @click="hello"> 小div <br /> <a href="http://www.baidu.com" @click.prevent="hello">去百度</a> </div> </div>
|
上面的这两个嵌套 div 中,如果点击了内层的 div,则外层的 div 也会被触发;这种问题可以事件修饰符来完成:
1 2 3 4 5 6 7 8 9
| <div style="border: 1px solid red;padding: 20px;" v-on:click.once="hello"> 大div <div style="border: 1px solid blue;padding: 20px;" @click.stop="hello"> 小div <br /> <a href="http://www.baidu.com" @click.prevent.stop="hello">去百度</a> </div> </div>
|
关于事件修饰符:
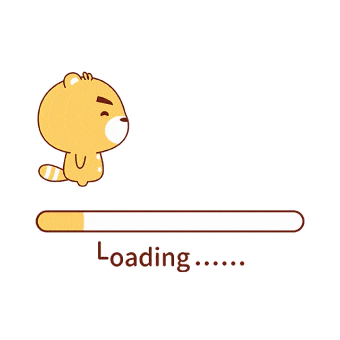
按键修饰符:
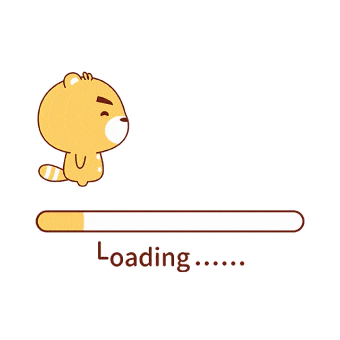
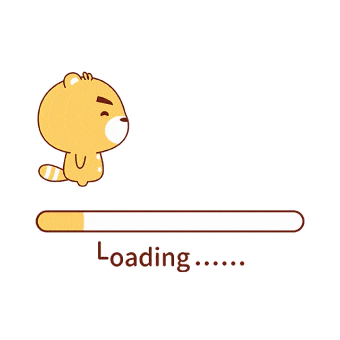
7、v-for 遍历循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head>
<body> <div id="app"> <ul> <li v-for="(user,index) in users" :key="user.name" v-if="user.gender == '女'" > 当前索引:{{index}} ==> {{user.name}} ==> {{user.gender}} ==>{{user.age}} <br />
对象信息: <span v-for="(v,k,i) in user">{{k}}=={{v}}=={{i}};</span> </li> </ul>
<ul> <li v-for="(num,index) in nums" :key="index"></li> </ul> </div> <script src="../node_modules/vue/dist/vue.js"></script> <script> let app = new Vue({ el: "#app", data: { users: [ { name: "柳岩", gender: "女", age: 21 }, { name: "张三", gender: "男", age: 18 }, { name: "范冰冰", gender: "女", age: 24 }, { name: "刘亦菲", gender: "女", age: 18 }, { name: "古力娜扎", gender: "女", age: 25 }, ], nums: [1, 2, 3, 4, 4], }, }); </script> </body> </html>
|
4、遍历的时候都加上:key 来区分不同数据,提高 vue 渲染效率
过滤器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head>
<body> <div id="app"> <ul> <li v-for="user in userList"> {{user.id}} ==> {{user.name}} ==> {{user.gender == 1?"男":"女"}} ==> {{user.gender | genderFilter}} ==> {{user.gender | gFilter}} </li> </ul> </div> <script src="../node_modules/vue/dist/vue.js"></script>
<script> Vue.filter("gFilter", function (val) { if (val == 1) { return "男~~~"; } else { return "女~~~"; } });
let vm = new Vue({ el: "#app", data: { userList: [ { id: 1, name: "jacky", gender: 1 }, { id: 2, name: "peter", gender: 0 }, ], }, filters: { genderFilter(val) { if (val == 1) { return "男"; } else { return "女"; } }, }, }); </script> </body> </html>
|
组件化
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head>
<body> <div id="app"> <button v-on:click="count++">我被点击了 {{count}} 次</button>
<counter></counter> <counter></counter> <counter></counter> <counter></counter> <counter></counter> <button-counter></button-counter> </div> <script src="../node_modules/vue/dist/vue.js"></script>
<script> Vue.component("counter", { template: `<button v-on:click="count++">我被点击了 {{count}} 次</button>`, data() { return { count: 1, }; }, });
const buttonCounter = { template: `<button v-on:click="count++">我被点击了 {{count}} 次~~~</button>`, data() { return { count: 1, }; }, };
new Vue({ el: "#app", data: { count: 1, }, components: { "button-counter": buttonCounter, }, }); </script> </body> </html>
|
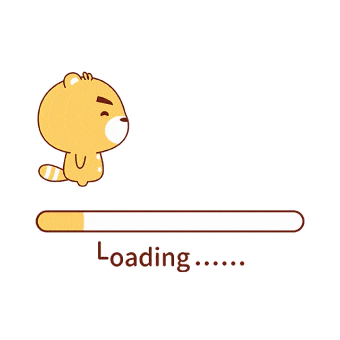
生命周期钩子函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head>
<body> <div id="app"> <span id="num">{{num}}</span> <button @click="num++">赞!</button> <h2>{{name}},有{{num}}个人点赞</h2> </div>
<script src="../node_modules/vue/dist/vue.js"></script>
<script> let app = new Vue({ el: "#app", data: { name: "张三", num: 100, }, methods: { show() { return this.name; }, add() { this.num++; }, }, beforeCreate() { console.log("=========beforeCreate============="); console.log("数据模型未加载:" + this.name, this.num); console.log("方法未加载:" + this.show()); console.log("html模板未加载:" + document.getElementById("num")); }, created: function () { console.log("=========created============="); console.log("数据模型已加载:" + this.name, this.num); console.log("方法已加载:" + this.show()); console.log("html模板已加载:" + document.getElementById("num")); console.log( "html模板未渲染:" + document.getElementById("num").innerText ); }, beforeMount() { console.log("=========beforeMount============="); console.log( "html模板未渲染:" + document.getElementById("num").innerText ); }, mounted() { console.log("=========mounted============="); console.log( "html模板已渲染:" + document.getElementById("num").innerText ); }, beforeUpdate() { console.log("=========beforeUpdate============="); console.log("数据模型已更新:" + this.num); console.log( "html模板未更新:" + document.getElementById("num").innerText ); }, updated() { console.log("=========updated============="); console.log("数据模型已更新:" + this.num); console.log( "html模板已更新:" + document.getElementById("num").innerText ); }, }); </script> </body> </html>
|
二、 element ui
官网: https://element.eleme.cn/#/zh-CN/component/installation
安装
在 main.js 中写入以下内容:
1 2 3 4
| import ElementUI from 'element-ui' import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
|