一、反射的概念
1、反射的理解
Reflection(反射) 是被视为动态语言的关键,反射的机制允许程序在执行期间借助 Reflection API 取得任何类的内部信息,并能直接操作任意对象的内部属性方法。
框架 = 反射 + 注解 +设计模式
2、反射的”动态性”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| @Test public void test1(){ for(int i = 0; i < 100; i++){ int num = new Random().nextInt(3); String classPath = ""; switch (num){ case 0: classPath = "java.util.Date"; break; case 1: classPath = "java.lang.object"; break; case 2: classPath = "com.oy.online.reflect"; break; } try { Object obj = getInstance(classPath); System.out.println(obj); } catch (Exception e) { e.printStackTrace(); } } }
public Object getInstance(String classPath) throws Exception { Class clazz = Class.forName(classPath); return clazz.newInstance(); }
|
3、反射机制提供的功能
- 在运行时判断一个对象所属的类
- 在运行时构造任意一个类的对象
- 在运行时判断一个类所具有的成员变量和方法
- 在运行时获取泛型信息
- 在运行时调用任意一个对象的成员变量和方法
- 在运行时处理注解
- 生成动态代理
4、相关 AIP
- java.lang.Class: 反射的源头
- java.lang.reflect.Method
- java.lang.reflect.Field
- java.lang.reflect.Consructor
二、Class 类的理解和获取 Class 实例
1、Class 类的理解
类的加载过程:
程序经过 javac.exe 命令以后,会生成一个多字节码文件(.class 结尾),接着我们使用 java.exe 对某个字节码文件进行解释运行。相当于将某个字节码文件记载到内存中。此过程就称为类的加载。加载到内存中的类,我们称为运行时类,就作为 Class 的一个实例。
class 的实例就对应着一个运行时类。
加载到内存中的运行时类,会缓存一定的时间,在此时间之内,我们可以通过不同的方式类获取运行类。
2、获取 Class 实例的方式
方式一:调用运行时类的属性:.class
1 2
| Class<Person> clazz = Person.class; System.out.println(clazz);
|
方式二:通过运行时类的对象,调用 getClass()
1 2 3
| Person p1 = new Person(); Class clazz = p1.getClass(); System.out.println(clazz);
|
方式三:调用 Class 的静态方法:forName(String classPath)
1 2
| Class<?> clazz = Class.forName("com.oy.online.bean.Person"); System.out.println(clazz);
|
方式四:使用类的加载器:ClassLoader
1 2 3
| ClassLoader classLoader = ReflectTest2.class.getClassLoader(); Class<?> clazz = classLoader.loadClass("com.oy.online.bean.Person"); System.out.println(clazz);
|
3、Class 实例化的结构
- class:外部类、成员(成员内部类,静态内部类)、局部内部类、匿名内部类
- interface:接口
- []: 数组
- enum: 枚举
- annotation: 注解@interface
- primitive type : 基本数据类型
- viod
三、了解 ClassLoad
1、类的加载过程
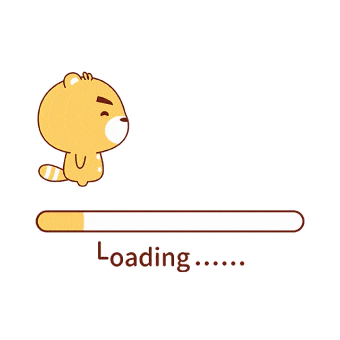
2、类的加载器的作用
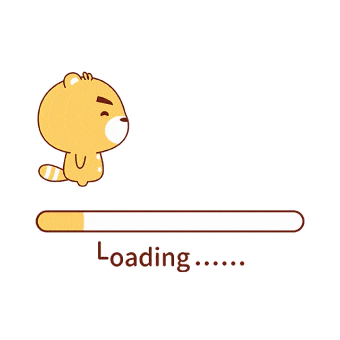
3、类的加载器的分类
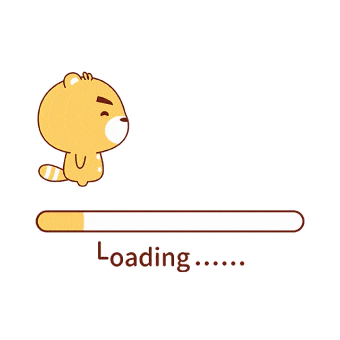
4、Java 类编译、运行的执行的流程
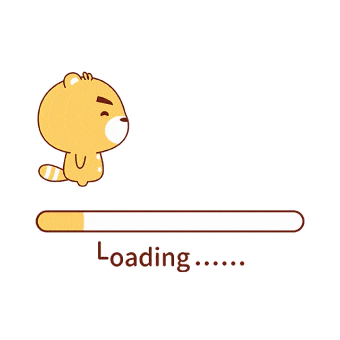
5、试用 Classloader 加载 src 目录下的配置文件
代码示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Test public void test5() throws Exception { Properties pros = new Properties();
ClassLoader clazz = ReflectTest2.class.getClassLoader(); InputStream is = clazz.getResourceAsStream("jdbc.properties"); pros.load(is);
String user = pros.getProperty("user"); String password = pros.getProperty("password"); System.out.println("user ="+ user + ",password ="+ password); }
|
四、反射应用一:创建运行时类的对象
1 2 3
| Class<Person> clazz = Person.class; Person obj = clazz.newInstance(); System.out.println(obj);
|
说明:
newInstance(): 调用此方法的运行类的对象。内部调用了运行类的空参的构造器。
要想方法正常的创建运行时类的对象,要求:
- 运行时必须是提供空参构造器
- 空参的构造器的访问权限的够。通常,设置为 public.
在 javabean 中要求提供一个 public 的空参构造器。原因:
- 便于通过反射,创建运行时类的对象
- 便于子类继承此运行类,默认调用 super()时,保证父类此构造器
五、反射应用二:获取运行时类的完整结构
通过反射,获取对应的运行时类中所有的属性、方法、构造器、父类、接口、父类的泛型、包、注解、异常等…
- getFields():获取当前运行时类及其父类中声明为 public 访问权限的属性
- getDeclaredFields():获取当前运行类中声明的属性。(不包含父类中声明的属性)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| @Test public void test2(){ Class<Person> clazz = Person.class;
Field[] fields = clazz.getFields(); for (Field f : fields) { System.out.println(f); }
System.out.println();
Field[] declaredFields = clazz.getDeclaredFields(); for (Field f : declaredFields) { System.out.println(f); } }
|
- getMethods(): 获取当前运行时类及其所父类中声明为 public 权限的方法
- getDeclaredMethods():获取当前运行时类中声明的所方法。(不包含父类中声明的方法)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @Test public void test3(){ Class<Person> clazz = Person.class;
Method[] methods = clazz.getMethods(); for (Method m : methods) { System.out.println(m); } System.out.println("***********************"); Method[] declaredMethods = clazz.getDeclaredMethods(); for (Method f : declaredMethods) { System.out.println(f); } }
|
- getConstructors(): 获取当前运行时类中声明为 public 的构造器
- getDeclaredConstructors(): 获取当前运行时类中声明的所的构造器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| @Test public void test4(){ Class<Person> clazz = Person.class; Constructor<?>[] constructors = clazz.getConstructors(); for (Constructor<?> c : constructors) { System.out.println(c); }
System.out.println("**************************");
Constructor<?>[] declaredConstructors = clazz.getDeclaredConstructors(); for (Constructor<?> d : declaredConstructors) { System.out.println(d); } }
|
- getSuperclass():获取运行时类的父类
1 2 3 4 5 6
| @Test public void test5(){ Class<Person> clazz = Person.class; Class<? super Person> superclass = clazz.getSuperclass(); System.out.println(superclass); }
|
- getGenericSuperclass(): 获取运行时类的带泛型的父类
1 2 3 4 5 6
| @Test public void test6(){ Class<Person> clazz = Person.class; Type genericSuperclass = clazz.getGenericSuperclass(); System.out.println(genericSuperclass); }
|
1 2 3 4 5 6 7 8 9
| @Test public void test7(){ Class<Person> clazz = Person.class; Type genericSuperclass = clazz.getGenericSuperclass(); ParameterizedType paramType = (ParameterizedType) genericSuperclass; Type[] actualTypeArguments = paramType.getActualTypeArguments(); System.out.println(((Class)actualTypeArguments[0]).getName()); }
|
- getInterfaces(): 获取运行时类实现的接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Test public void test8(){ Class<Person> clazz = Person.class;
Class<?>[] interfaces = clazz.getInterfaces(); for (Class<?> i : interfaces) { System.out.println(i); }
System.out.println("****************"); Class<?>[] interfaces1 = clazz.getSuperclass().getInterfaces(); for (Class<?> c : interfaces1) { System.out.println(c); }
|
1 2 3 4 5 6
| @Test public void test9(){ Class<Person> clazz = Person.class; Package pack = clazz.getPackage(); System.out.println(pack); }
|
- getAnnotations(): 获取运行时类声明的注解
1 2 3 4 5 6 7
| @Test public void test10(){ Class<Person> clazz = Person.class; Annotation[] annotations = clazz.getAnnotations(); for (Annotation a : annotations) { System.out.println(a); }
|
六、反射应用三:调用运行时类的指定结构
1、调用指定的属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Test public void test1() throws Exception { Class<Person> clazz = Person.class;
Person p = clazz.newInstance();
Field name = clazz.getDeclaredField("name");
name.setAccessible(true);
name.set(p,"Tom");
System.out.println(name.get(p)); }
|
2、调用指定的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| @Test public void test2() throws Exception { Class<Person> clazz = Person.class;
Person p = clazz.newInstance();
Method show = clazz.getDeclaredMethod("show", String.class); show.setAccessible(true);
Object returnValue = show.invoke(p, "CHN"); System.out.println(returnValue);
System.out.println("********************");
Method showDesc = clazz.getDeclaredMethod("showDesc"); showDesc.setAccessible(true); Object returnVal = showDesc.invoke(Person.class); System.out.println(returnVal); }
|
3、调用指定的构造器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Test public void test3() throws Exception { Class<Person> clazz = Person.class;
Constructor<Person> constructor = clazz.getDeclaredConstructor(String.class);
constructor.setAccessible(true);
Person per = constructor.newInstance("Tom"); System.out.println(per); }
|
七、反射应用四:动态代理
1、代理模式的原理
使用一个代理将对象包装起来,然后用该代理对象去掉原始对象。任何对原始对象的调用都要通过代理。代理对象决定是否以及何时将方法调用转到原始对象上。
2、静态代理
- 举例
1 2 3 4 5 6 7 8
| 实现Runnable接口的方法创建多线程。 Class MyThread implements Runnable{} Class Thread implements Runnable{} main(){ MyTread t = new MyThread(); Thread thread = new Thread(t); thresd.start(); }
|
- 静态代理的缺点
- 代理类和目标对象的类都是在编译间确定下来的,不利于程序的扩展。
- 每一个代理类只能为一个接口服务,这样一来程序开发中必然产生过多的代理。
动态代理的特点
动态代理是指客户端通过代理类来调用其他对象的方法,并且是在程序运行时根据需要动态创建目标类的代理对象
3、动态代理的实现
需要解决的两个主要问题:
问题一:如何根据加载到内存中的被代理类,动态的创建一个代理类及其对象。(通过 Proxy.newProxyInstance()实现)
问题二:当通过代理类的对象调用方法 a 时,如何动态的去调用被代理类的同名方法 a。(通过 InvocationHandler 接口的实现类及其方法 invoke())
代码示例
1 2 3 4 5 6 7
| interface Human{
String getBelief();
void eat(String food);
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class SuperMan implements Human{
@Override public String getBelief() { return "I believe I can fly!"; }
@Override public void eat(String food) { System.out.println("我喜欢吃" + food); } }
|
1 2 3 4 5 6 7 8 9 10 11 12
| class HumanUtil{
public void method1(){ System.out.println("====================通用方法一====================");
}
public void method2(){ System.out.println("====================通用方法二===================="); }
}
|
1 2 3 4 5 6 7 8 9 10 11
| class ProxyFactory{ public static Object getProxyInstance(Object obj){ MyInvocationHandler handler = new MyInvocationHandler();
handler.bind(obj);
return Proxy.newProxyInstance(obj.getClass().getClassLoader(),obj.getClass().getInterfaces(),handler); }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class MyInvocationHandler implements InvocationHandler{
private Object obj;
public void bind(Object obj){ this.obj = obj; }
@Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
HumanUtil util = new HumanUtil(); util.method1();
Object returnValue = method.invoke(obj,args);
util.method2();
return returnValue;
} }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class ProxyTest {
public static void main(String[] args) { SuperMan superMan = new SuperMan(); Human proxyInstance = (Human) ProxyFactory.getProxyInstance(superMan); String belief = proxyInstance.getBelief(); System.out.println(belief); proxyInstance.eat("四川麻辣烫");
System.out.println("*****************************");
NikeClothFactory nikeClothFactory = new NikeClothFactory();
ClothFactory proxyClothFactory = (ClothFactory) ProxyFactory.getProxyInstance(nikeClothFactory);
proxyClothFactory.produceCloth();
} }
|