一、InetAddress 类的使用
1、实现网络通信需要解决的两个问题
- 如何准确的定位网络上一台或多台的主机;定位主机上的特定应用
- 找到主机后如何高效地进行数据传输
2、网络通信的两个要素
- 对应问题一:IP 和端口号
- 对应问题二:提供网络协议:TCP/IP 参考模型(应用层、传输层、网络层、物理+数据链路层)
3、通信要素一:IP 和端口号
① IP 的解释
- 唯一的标识 Internet 上的计算机(通信载体)
- 在 Java 中使用 InternetAddress 类代表 IP
- IP 分类: IPV4 和 IPV6 ; 万维网 和 局域网
- 域名:www.baidu.com ;
- 域名解析:域名容易记忆。当在连接网络时输入一个主机的域名后,域名服务器(DNS)负责将域名转化为 IP 地址,这样才能和本地建立连接。– 域名解析
- 本地回路地址:127.0.0.1 对应着:localhost
② InetAddress 类
此类的一个对象就代表着一个具体的 IP 地址
- 实例化
1
| getByName(String host)、getLocalhost()
|
- 常用方法
1
| getHostName()/getHostAddress()
|
4、端口号
要求:不同的进程不同的端口号
范围:被规定为一个 16 位的整数 0~65535
端口号与 IP 地址的组合得出一个网络套接字:Socket
5、通信要素二:网络通信协议
① 分类模型
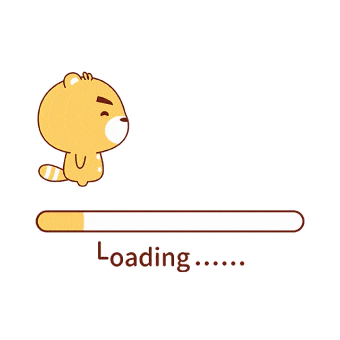
② TCP 和 UDP 的区别
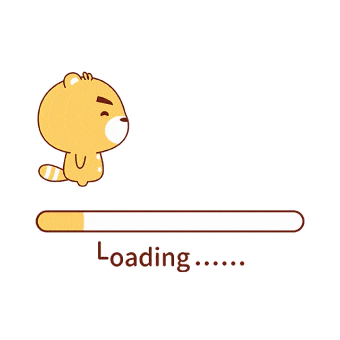
③ TCP 三次握手和四次挥手
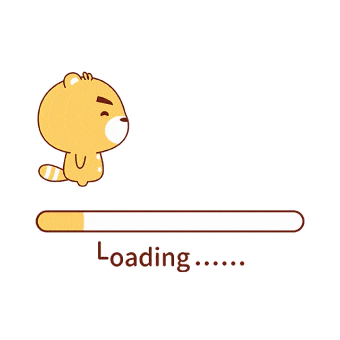
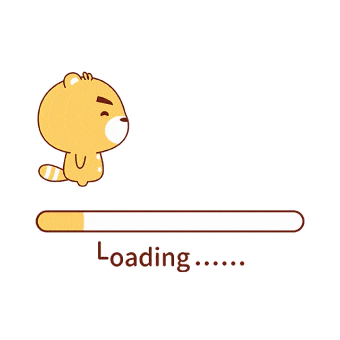
④ 补充
1 2 3 4 5 6 7 8
| InetAddress.getByName("192.168.12.67");
InetAddress.getLocalhost();
inet2.getHostName()
inet2.getHostAddress()
|
二、TCP 网络编程
代码示例:客户端发送信息给服务端,服务端将数据显示在控制台上
客户端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| @Test public void client(){ Socket socket = null; OutputStream os = null; try { InetAddress inet = InetAddress.getByName("127.0.0.1"); socket = new Socket(inet, 8899); os = socket.getOutputStream(); os.write("你好,我是客户端".getBytes()); } catch (IOException e) { e.printStackTrace(); } finally { try { if(os != null){
os.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(socket != null){
socket.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
服务端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
| @Test public void Server(){ ServerSocket serverSocket = null; Socket socket = null; InputStream is = null; ByteArrayOutputStream baos = null; try { serverSocket = new ServerSocket(8899); socket = serverSocket.accept(); is = socket.getInputStream();
baos = new ByteArrayOutputStream(); byte[] bt = new byte[1024]; int len; while((len = is.read(bt)) != -1){ baos.write(bt, 0, len); }
System.out.println(baos.toString()); System.out.println("收到了来自:"+socket.getInetAddress().getHostAddress()+"的数据"); } catch (IOException e) { e.printStackTrace(); } finally { try { if(serverSocket != null){
serverSocket.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(socket != null){
socket.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(is != null){
is.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(baos != null){
baos.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
代码示例 2:客户端发送文件给服务端,服务端将文件保存在本地。
客户端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| @Test public void client(){ Socket socket = null; OutputStream os = null; FileInputStream fis = null; try { socket = new Socket(InetAddress.getByName("127.0.0.1"), 8899); os = socket.getOutputStream();
fis = new FileInputStream("1.jpg"); byte[] bt = new byte[1024]; int len; while((len = fis.read(bt)) != -1){ os.write(bt, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { if(fis != null){
try { fis.close(); } catch (IOException e) { e.printStackTrace(); } } if(os != null){
try { os.close(); } catch (IOException e) { e.printStackTrace(); } } if(socket != null){
try { socket.close(); } catch (IOException e) { e.printStackTrace(); } } } }
|
服务端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| @Test public void Server(){ ServerSocket ss = null; Socket socket = null; InputStream is = null; FileOutputStream fos = null; try { ss = new ServerSocket(8899); socket = ss.accept(); is = socket.getInputStream(); fos = new FileOutputStream(new File("图片2.jpg"));
byte[] bt = new byte[1024]; int len; while((len = is.read(bt)) != -1){ fos.write(bt, 0,len); } } catch (IOException e) { e.printStackTrace(); } finally { if(ss != null){ try { ss.close(); } catch (IOException e) { e.printStackTrace(); }
} if(socket != null){ try { socket.close(); } catch (IOException e) { e.printStackTrace(); } } if(is != null){ try { is.close(); } catch (IOException e) { e.printStackTrace(); } } if(fos != null){ try { fos.close(); } catch (IOException e) { e.printStackTrace(); } } }
}
|
三、UDP 编程
发送端:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Test public void sender(){ DatagramSocket socket = null; try { socket = new DatagramSocket();
String str = "我是UDP方式发送的导弹"; byte[] data = str.getBytes(); InetAddress inet = InetAddress.getLocalHost(); DatagramPacket packet = new DatagramPacket(data, 0, data.length, inet, 9090);
socket.send(packet); } catch (IOException e) { e.printStackTrace(); } finally { socket.close(); } }
|
接收端:
1 2 3 4 5 6 7 8 9 10
| @Test public void receiver() throws IOException { DatagramSocket socket = new DatagramSocket(9090);
byte[] buffer = new byte[1024]; DatagramPacket packet = new DatagramPacket(buffer, 0, buffer.length);
socket.receive(packet); System.out.println(new String(packet.getData(),0,packet.getLength())); }
|
四、URL 编程
统一资源定位符,对应着互联网的某一资源地址
2、URL 的 5 个基本结构
3、实例化
1
| URL url = new URL("http://localhost:8080/examples/beauty.jpg?username=Tom");
|
4、常用方法
方法 | 描述 |
---|
public String getProtocol() | 获取该 URL 的协议名 |
public String getHost() | 获取 URL 的主机名 |
public String getPort() | 获取 URL 的端口号 |
public String getPath() | 获取 URL 的文件路径 |
public String getFile() | 获取 URL 的文件名 |
public String getQuery() | 获取 URL 的查询名 |
五、可以读取、下载对应的 url 资源
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| public static void main(String[] args) {
HttpURLConnection urlConnection = null; InputStream is = null; FileOutputStream fos = null; try { URL url = new URL("http://localhost:8080/examples/beauty.jpg");
urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.connect();
is = urlConnection.getInputStream(); fos = new FileOutputStream("day10\\beauty3.jpg");
byte[] buffer = new byte[1024]; int len; while((len = is.read(buffer)) != -1){ fos.write(buffer,0,len); }
System.out.println("下载完成"); } catch (IOException e) { e.printStackTrace(); } finally { if(is != null){ try { is.close(); } catch (IOException e) { e.printStackTrace(); } } if(fos != null){ try { fos.close(); } catch (IOException e) { e.printStackTrace(); } } if(urlConnection != null){ urlConnection.disconnect(); } } }
|