一、File 类的使用
1、File 类的理解
① File 类的一个对象,代表一个文件或文件目录(俗称:文件夹)
② File 类声明的砸 Java.io 包下
③ File 类中涉及关于文件或文件目录的创建、删除、重命名、修改时间、文件大小等方法,并涉及到写入的读取文件内容的操作。如果需要读取或写入的 ”终点“。
2、File 的实例化
常用的构造器
1 2 3
| File(String filePath) File(String parenPath, String childPath) File(File parenFile, String childPath)
|
路径的分类
1 2
| 相对路径:相对某个路径,指明的路径 绝对路径:包含盘符在内的文件或文件目录的路径
|
路径分隔符
1 2
| windows 和 DOC 系统的默认使用“\”来表示 UNIX 和 URL 使用“/” 来表示
|
二、流的分类
1、流的分类
- 操作数据的单位:字节流、字符流
- 数据的流向:输入流、输出流
- 流的角色:节点流、处理流
图示:
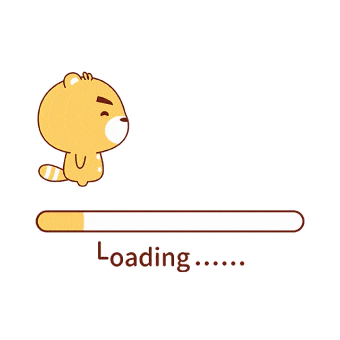
2、流的体系结构
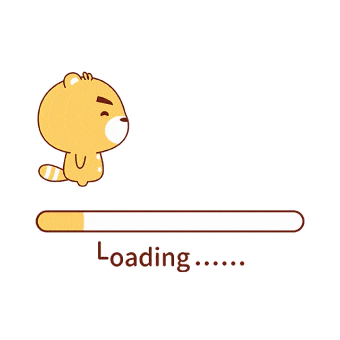
说明: 红框对应的是 IO 流中的 4 个抽象基类。
3、输入、输出的标准化过程
① 输入过程
- 创建 File 类的对象,指明读取数据的来源。(要求此文件一定存在)
- 创建相对应的输入流,将 File 类的对象作为参数,传入流的构造器中
- 具体的读入过程:
创建相对应的 byte[ ] 或 char[ ] - 关闭流资源
说明: 程序中出现的异常需要使用 try-catch-finally 处理。
② 输入过程
- 创建 File 类对象,指明写出的数据的位置。(不要求此文件一定存在)
- 创建相对应的输出流,将 File 类的对象作为参数,传入流的构造器中。
- 具体的写入过程:
write(byte[ ] 或 char[ ], 0, len) - 关闭流资源
说明:程序中出现的异常需要使用 try-catch-finally 处理。
三、节点流(或文件流)
1、FileReader/FileWirter 的使用
① FileReader 的使用
- read() 的理解:返回读入的一个字符。如果达到文件的末尾。返回-1
- 异常的处理:为了保证流的资源一定可以执行关闭操作。需要使用 try-catch-finally 处理
- 读入的文件一定要存在,否则就会报 FileNotExcption。
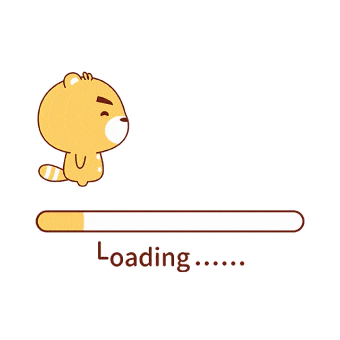
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| @Test public void test() { FileReader fr = null; try { File file = new File("hello.txt");
fr = new FileReader(file);
char[] ch = new char[1024]; int len; while((len = fr.read(ch)) != -1){ String str = new String(ch, 0, len); System.out.println(str); } } catch (IOException e) { e.printStackTrace(); } finally { try { if(fr != null){ fr.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
② FileWrite 的使用
输出操作,对应的 File 可以不存在的。并不会报异常
File 对应的硬盘中文件如果不存在,在输出的过程中,会自动创建此文件。
File 对应的硬盘中文件如果存在:
如果流使用的构造器是:FileWriter(file, false)/ FileWriter(file): 对原文件的覆盖
如果流使用的构造器是:FileWriter(file,true): 不会对原文件覆盖,而是原文件基础上追加内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| @Test public void test2() { FileWriter fw = null; try { File file = new File("hello.txt");
fw = new FileWriter(file, false);
fw.write("I have a dream!\n"); fw.write("you need to have a dream!"); } catch (IOException e) { e.printStackTrace(); } finally { try { if (fw != null){ fw.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
③ 文本文件复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| @Test public void test3(){ FileReader fr = null; FileWriter fw = null; try { File srcfile = new File("hello.txt"); File dsrcfile1 = new File("hello1.txt");
fr = new FileReader(srcfile); fw = new FileWriter(dsrcfile1);
char[] ch = new char[1024]; int len; while((len = fr.read(ch)) != -1){ fw.write(ch,0,len); } } catch (IOException e) { e.printStackTrace(); } finally { try { if(fw != null){
fw.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(fr != null){
fr.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
- 对于文本文件(**.txt**, .java, .c, .cpp), 使用字符流处理
- 对于非文本文件(**.jpg** , .mp3, .mp4, .avi, .doc, .ppt),使用字节流处理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| @Test public void test1(){ FileInputStream fis = null; FileOutputStream fos = null; try { File srcfile = new File("图片.jpg"); File desrfile = new File("图片2.jpg");
fis = new FileInputStream(srcfile); fos = new FileOutputStream(desrfile);
byte[] bt = new byte[1024]; int len; while((len = fis.read(bt)) != -1){ fos.write(bt,0,len); } } catch (IOException e) { e.printStackTrace(); } finally { try { if(fis != null){ fis.close();
} } catch (IOException e) { e.printStackTrace(); } try { if(fos != null){
fos.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
【注意】
- IDEA:
- 如果使用单元测试方法,相对路径当前 Module 的。
- 如果使用 main() 测试,相对路径基于当前 Project 的。
- Eclipes:
- 单元测试方法还是 main(), 相对路径都是基于当前 Project 的。
四、缓冲流
1、缓冲流涉及到的类
- BufferedInputStream
- BufferedOutputStream
- BufferedReader
- BufferedWriter
2、作用
作用:提高流的读取、写入的速度
提高读写速度的原因:内部提供了一个缓冲区。默认情况下是 8kb
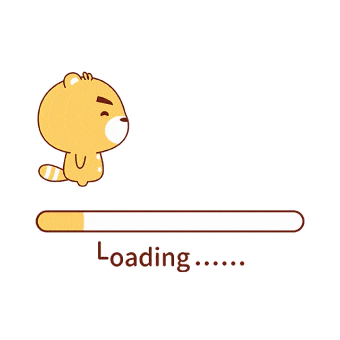
3、代码演示
① 使用 BufferadInputStream 和 BufferadOutputStream :处理非文本
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| @Test public void test1(){ BufferedInputStream bis = null; BufferedOutputStream bos = null; try { File srcfile = new File("图片.jpg"); File dsrcfile = new File("图片3.jpg");
FileInputStream fis = new FileInputStream(srcfile); FileOutputStream fos = new FileOutputStream(dsrcfile); bis = new BufferedInputStream(fis); bos = new BufferedOutputStream(fos);
byte[] bt = new byte[1024]; int len; while((len = bis.read(bt)) != -1){ bos.write(bt, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { try { bos.close(); } catch (IOException e) { e.printStackTrace(); } try { bis.close(); } catch (IOException e) { e.printStackTrace(); } }
}
|
② 使用 BufferedReader 和 BufferedWriter:处理文本文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| @Test public void test2(){ BufferedReader br = null; BufferedWriter bw = null; try { File srcfile = new File("hello.txt"); File dsrcfile = new File("hello3.txt");
br = new BufferedReader(new FileReader(srcfile)); bw = new BufferedWriter(new FileWriter(dsrcfile));
String data; while((data = br.readLine()) != null){ bw.write(data); bw.newLine(); } } catch (IOException e) { e.printStackTrace(); } finally { try { br.close(); } catch (IOException e) { e.printStackTrace(); } try { bw.close(); } catch (IOException e) { e.printStackTrace(); } } }
|
1 2 3 4 5 6 7
| char[] cbuf = new char[1024]; int len; while((len = br.read(cbuf)) != -1){ bw.write(cbuf,0,len); bw.flush(); }
|
五、转换流
1、转换流涉及到的类:属于字符流
- InputStreamReader: 将一个字节的输入转换为字符的输入流
- OutputStreamWriter: 将一个字符的输入流转换字节的输出流
- 说明:编码决定了解码的的方式
2、方式
提供字节流与字符流之间的转换
3、图示
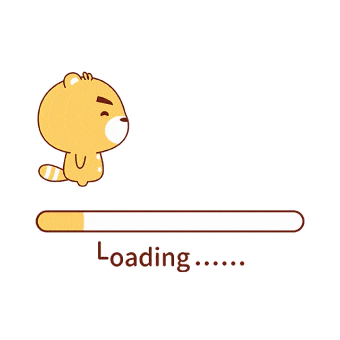
4、典型案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| @Test public void test1() { InputStreamReader isr = null; try { FileInputStream fis = new FileInputStream("hello.txt"); isr = new InputStreamReader(fis, "UTF-8");
char[] ch = new char[1024]; int len; while((len = isr.read(ch)) != -1){ String str = new String(ch, 0, len); System.out.println(str); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (isr != null){
isr.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
- 处理异常使用 try-catch
- 综合使用 InputStreamReader 和 OutputStreamWriter
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| @Test public void test2() { InputStreamReader isr = null; OutputStreamWriter osw = null; try { File file1 = new File("hello.txt"); File file2 = new File("hello_gbk.txt");
FileInputStream fis = new FileInputStream(file1); FileOutputStream fos = new FileOutputStream(file2);
isr = new InputStreamReader(fis, "UTF-8"); osw = new OutputStreamWriter(fos, "gbk");
char[] ch = new char[1024]; int len; while((len = isr.read(ch)) != -1){ osw.write(ch, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { try { if(isr != null){
isr.close(); } } catch (IOException e) { e.printStackTrace(); } try { if(osw != null){
osw.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
六、对象流
1、对象流
- ObjectInputStream 和 ObjectOutputStream
2、作用
ObjectInputStream: 内存中的对象 –> 存储中的文件、通过网路传输过去:序列化过程
ObjectOutputStream: 存储中文件、通过网络接收过来 –> 内存中的对象:反序列化的过程
3、对象的序列化机制
- 对象序列化机制允许把内存中的 Java 对象转换成平台无关的二进制流,从而允许把这二进制流持久保存在硬盘上,或通过网路将这种二进制流输入到另一个网络节点
- 当其他程序获取到这种二进制流,就可以恢复原来的 java 对象
4、序列化代码
创建 Person 类(实现 Serializable 接口,添加序列号):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| public class Person implements Serializable {
public static final long serialVersionUID = 475463534532L; private String name; private int id;
public Person() { }
public Person(String name, int id) { this.name = name; this.id = id; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
@Override public String toString() { return "Person{" + "name='" + name + '\'' + ", id=" + id + '}'; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| @Test public void test1() { ObjectOutputStream oos = null; try { oos = new ObjectOutputStream(new FileOutputStream("object.dat")); oos.writeObject(new String("你好")); oos.flush(); oos.writeObject(new Person("小明",23)); oos.flush(); } catch (IOException e) { e.printStackTrace(); } finally { try { if (oos != null){
oos.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
5、反序列化代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| @Test public void test2(){ ObjectInputStream ois = null; try { ois = new ObjectInputStream(new FileInputStream("object.dat")); Object obj = ois.readObject(); String str = (String) obj; Person p = (Person) ois.readObject();
System.out.println(str); System.out.println(p); } catch (IOException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } finally { try { if (ois != null){
ois.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
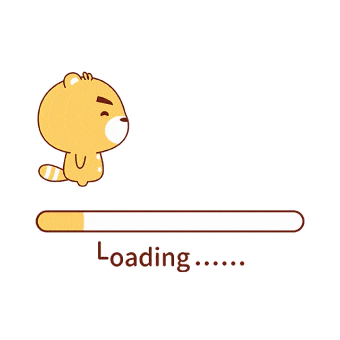
6、实现序列化的对象所属的类需要满足:
- 需要实现接口:Serializable
- 当前类提供一个全局常量:SeriaVersionUID
- 除了当前 Person 类需要实现 Serializable 接口之外,还需要保证其内部类所属性也必须是可序列化。(默认情况下,基本数据类型可序列化)
- ObjectOutputStream 和 ObjectInputStream 不能序列化 static 修饰的成员变量。
七、其他流的使用
1、标准输入输出流
- System.in : 标准的输入流,默认从键盘输入
- System.out : 标准的输出流,默认从控制台输出
修改默认的输入和输出行为:
System 类的 setIn(InputStream is) / setOut(prinStream ps) 方式重新指定输入和输出流。
2、打印流
- PrinStream 和 PrintWriter
- 说明:
- 提供一系列重载的 print()的方法,用于多种数据类型的输出
- System.out 返回的是 PrintStream 的实例
3、数据流
- DataInputStream 和 DataOutputStream
作用:用于读取或写出基本数据类型的变量或字符串
实例 1:将内存中的字符串、基本数据类型的变量写到文件中。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| @Test public void test1(){
DataOutputStream dos = null; try { dos = new DataOutputStream(new FileOutputStream("data.txt"));
dos.writeUTF("OY"); dos.flush(); dos.writeInt(19); dos.flush(); dos.writeBoolean(true); dos.flush(); } catch (IOException e) { e.printStackTrace(); } finally { try { if(dos != null){
dos.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
实例 2:将文件中存储的基本数据类型变量和字符串读取到内存中,保存在变量中。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| @Test public void test2() { DataInputStream dis = null; try { dis = new DataInputStream(new FileInputStream("data.txt")); String name = dis.readUTF(); int age = dis.readInt(); Boolean isMale = dis.readBoolean();
System.out.println("name=" + name); System.out.println("age=" + age); System.out.println("isMale=" + isMale); } catch (IOException e) { e.printStackTrace(); } finally { try { if(dis != null){
dis.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
八、RandomAccesFile 的使用
1、随机存储文件流
- RondomAccessFile 直接继承于 java.Object 类,实现 DataInput 和 DataOutput 接口
- RandomAccessFile 既可以作为输入流,又可以作为一个输出流
- 如果 RandomAccessFile 作为输出流时,写出到的文件如果不存在,则在执行的过程中自动创建。
- 如果写出到的文件存在,则也对原文件内容进行覆盖。(默认情况下,从头覆盖)
- 可以通过相关的操作,实现 RandomAccessFile”插入”数据的效果。seek(int pos)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| @Test public void test1(){ RandomAccessFile raf1 = null; RandomAccessFile raf2 = null; try { raf1 = new RandomAccessFile(new File("图片.jpg"), "r"); raf2 = new RandomAccessFile(new File("图片4.jpg"), "rw");
byte[] bt = new byte[1024]; int len; while((len = raf1.read(bt)) != -1){ raf2.write(bt, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (raf1 != null){
raf1.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (raf2 != null){
raf2.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|