一、ElasticSearch 安装环境
二、安装步骤
① 下载 docker ElasticSearch 容器镜像
Docker Hub 镜像下载地址:https://hub.docker.com/
1
| docker pull elasticsearch:7.6.2
|
② 启动镜像映射
1
| docker run -e ES_JAVA_OPTS="-Xms256m -Xmx256m" -d -p 9200:9200 -p 9300:9300 --name ES01 elasticsearch:7.6.2
|
注意:根据自己情况来配置 ==-e ES_JAVA_OPTS=”-Xms256m -Xmx256m”== 不配置的话,启动会占用你的 2G 内存,反之,配置的话,启动则根据你配置的内存来分配。
异常:如果启动后,docker 容器自动关闭,且无法访问
1
| docker logs -f id[容器id] // 查看启动日志
|
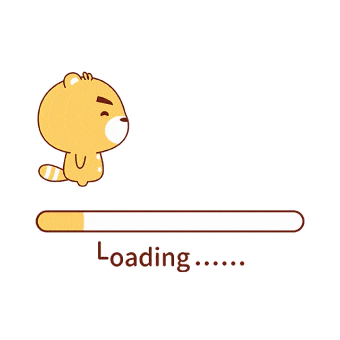
1 2 3 4 5 6 7 8 9
| // 修改 elasticsearch.yml 配置即可解决 // 先查找 elasticsearch.yml find / -name elasticsearch.yml vim elasticsearch.yml 路径
// 在elasticsearch.yml添加下面内容 bootstrap.system_call_filter: false cluster.initial_master_nodes: ["node-1"]
|
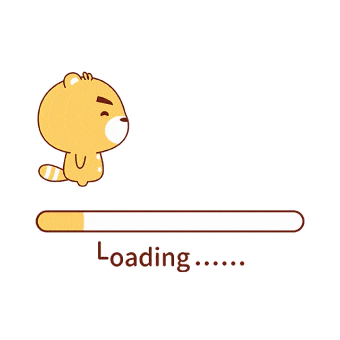
解决详细方法,请参考博客
1
| sysctl -w vm.max_map_count=262144
|
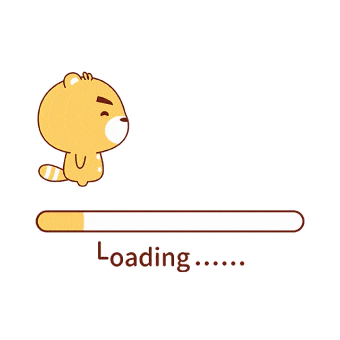
1 2
| //重新启动容器 docker start 容器[id]
|
③ 测试
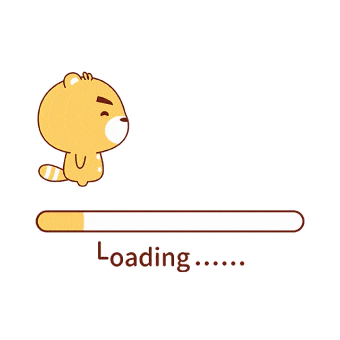
三、ElasticSearch 基本语法
请参考官方文档进行学习
简单示例演示:使用工具: Postman
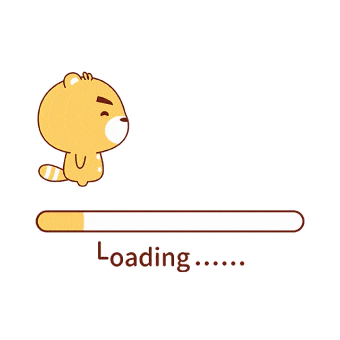
发送成功:
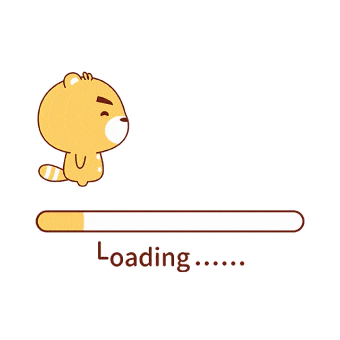
注意:如果 put 出现 503 错误,需要在配置elasticsearch.yml文件中添加
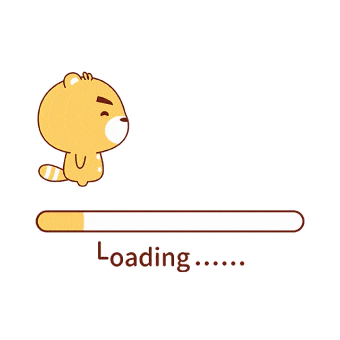
查询:
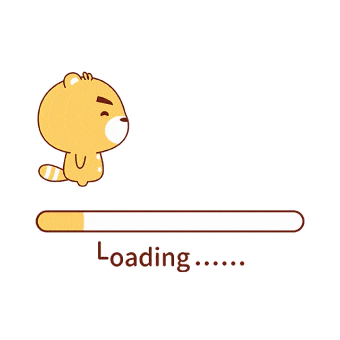
四、整合 ElasticSearch
springBoot 2.3.0 版本及以后版本不支持 es 查询工具 jestClient 自动注入
① Jest
2.2 版本
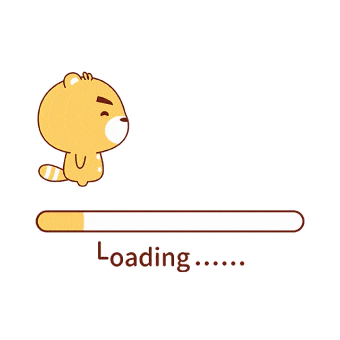
1.引入 jest
1 2 3 4 5
| <dependency> <groupId>io.searchbox</groupId> <artifactId>jest</artifactId> <version>6.3.1</version> </dependency>
|
2. application.yml 配置
1
| spring.elasticsearch.jest.uris=http://192.168.64.129:9200
|
3.创建 bean
1 2 3 4 5 6 7 8 9 10
| public class Article {
@JestId private Integer id; private String author; private String title; private String content;
}
|
4.测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| @Autowired private JestClient jestClient; @Test void contextLoads() {
Article article = new Article(); article.setId(1); article.setTitle("三国演义"); article.setAuthor("罗贯中"); article.setContent("Hello World");
Object source; Index index = new Index.Builder(article).index("sanguo").type("news").build();
try { jestClient.execute(index); } catch (IOException e) { e.printStackTrace(); } }
|
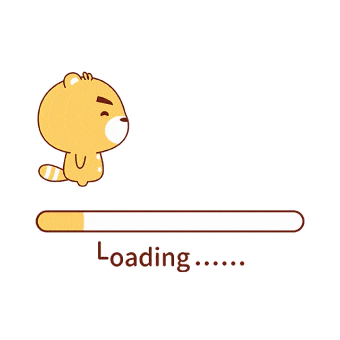
2.3 版本
由于 springboot 2.3.0 以后版本不支持自动注入 JestClient,如下图我们在 yml 文件中配置 JestClient 时会出现划掉的线提示。我们采取手动配置的方式
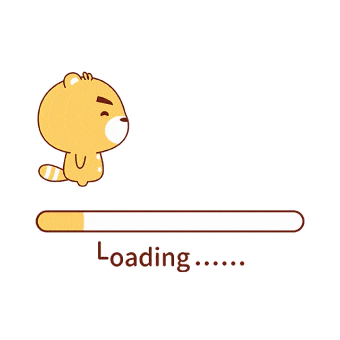
1.引入 jest
1 2 3 4 5
| <dependency> <groupId>io.searchbox</groupId> <artifactId>jest</artifactId> <version>6.3.1</version> </dependency>
|
2.创建 bean
1 2 3 4 5 6 7 8 9 10
| public class Article {
@JestId private Integer id; private String author; private String title; private String content;
}
|
3.手动注入和测试
【jestClient.java】
1 2 3 4 5 6 7 8 9 10 11
| @Repository public class jestClient { public JestClient getJestCline(){ JestClientFactory factory = new JestClientFactory(); factory.setHttpClientConfig(new HttpClientConfig .Builder("http://192.168.64.129:9200") .multiThreaded(true) .build()); return factory.getObject(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Test void contextLoads() { Article article = new Article(); article.setId(1); article.setTitle("西游记"); article.setAuthor("吴承恩"); article.setContent("Hello World"); Index index = new Index.Builder(article).index("xiyou").type("news").build();
try { jestClient.getJestCline().execute(index); } catch (IOException e) { e.printStackTrace(); } }
|
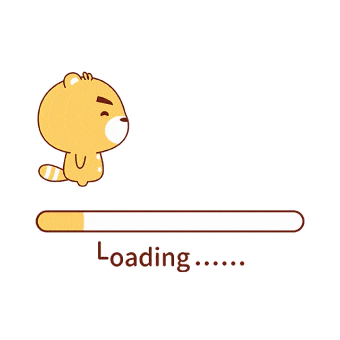
4.表达式测试搜索(2.2 和 2.3)
更多操作:https://github.com/searchbox-io/Jest/tree/master/jest
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| @Test public void search(){ String json ="{\n" + " \"query\" : {\n" + " \"match\" : {\n" + " \"content\" : \"hello\"\n" + " }\n" + " }\n" + "}"; Search search = new Search.Builder(json).addIndex("xiyou").addType("news").build();
try { SearchResult result = jestClient.getJestCline().execute(search); System.out.println(result); } catch (IOException e) { e.printStackTrace(); } }
|
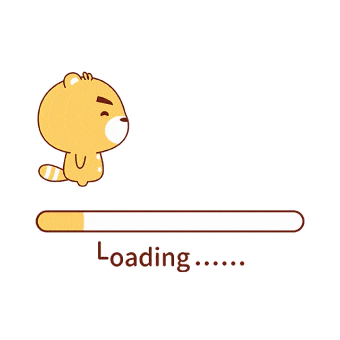
② springDataElasticSearch
2.2 版本
1.application.yml 配置
1 2
| spring.data.elasticsearch.cluster-name=elasticsearch spring.data.elasticsearch.cluster-nodes=118.24.44.169:9301
|
其他的不过多的赘述了,不在向上面分版本,其他的参考 2.3 版本,可自行百度。
2.3 版本
1.引入 spring-boot-starter-data-elasticsearch
1 2 3 4
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-elasticsearch</artifactId> </dependency>
|
2.安装 Spring Data 对应版本的 ElasticSearch
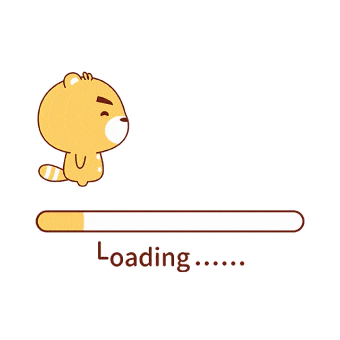
版本适配说明:https://github.com/spring-projects/spring-data-elasticsearch
如果版本不适配:
1)、升级 SpringBoot 版本
2)、安装对应版本的 ES
3.手动配置 Client
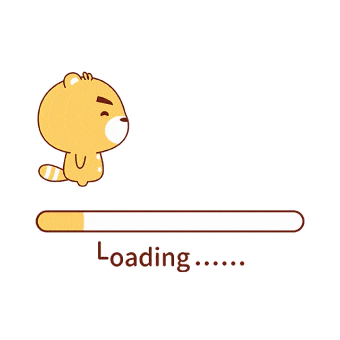
现在 spring 官方推荐我们用High Level REST Client
来配置
手动配置如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| @Configuration public class ElasticSearchConfig extends AbstractElasticsearchConfiguration {
@Override public RestHighLevelClient elasticsearchClient() { ClientConfiguration configuration = ClientConfiguration.builder( ) .connectedTo("192.168.64.129:9200") .build(); RestHighLevelClient client = RestClients.create(configuration).rest(); return client; } }
|
4.创建 bean
1 2 3 4 5 6 7 8 9
| @Document(indexName = "at") public class Book { private Integer id; private String bookname; private String author;
}
|
5.操作 ES 有两大类
- Elasticsearch Repositories
- Elasticsearch Operations
Elasticsearch Repositories
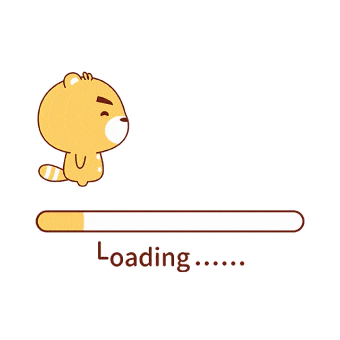
先用Elasticsearch Repositories
,注意接口与接口的关系是 extends
1 2 3 4 5
| @Repository public interface Bookrepository extends ElasticsearchRepository<Book,Integer> { List<Book> findBookById(int i); }
|
Elasticsearch Repositories
提供了许多关键字,来帮助我们实现方法。我们只需要写抽象方法即可,Elasticsearch Repositories
会根据方法名自动我们为我们实现,比如上面find
和By
就是关键字。我们需要在 springboot 主配置类上加上注解@EnableElasticsearchRepositories
可以使用 Elasticsearch 提供的的关键字(方法)列表,常用关键字如下
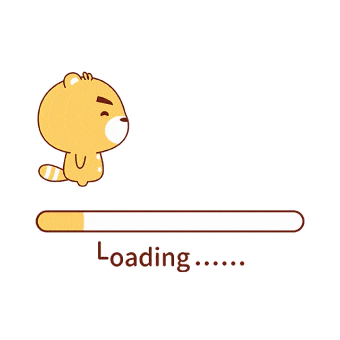
然后测试一下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| @SpringBootTest class ElasticsearchApplicationTests {
@Autowired Bookrepository bookrepository;
@Test void contextLoads() { Book book=new Book(1,"西游记","吴承恩"); bookrepository.save(book); }
@Test void testRepositories(){ List<Book> bookById = bookrepository.findBookById(1); System.out.println(bookById.get(0)); }
}
|
结果
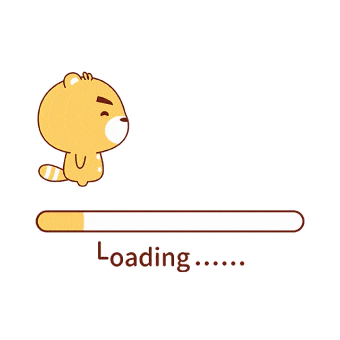
Elasticsearch Operations
ElasticsearchTemplate 是基于Transport client
的,Transport client
将会再 ES8.0 中被弃用,用谁不用我多说了吧。
使用 Elasticsearch Operations 我们需要修改上面的配置类,需要继承AbstractElasticsearchConfiguration
,因为基类AbstractElasticsearchConfiguration
已经提供了ElasticsearchRestTemplate
这个bean
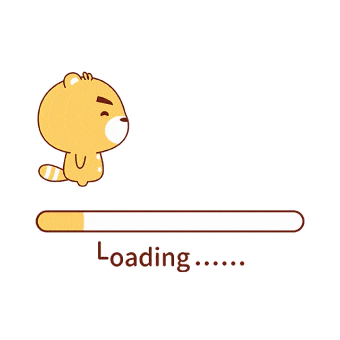
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| @Configuration public class RestClientConfig extends AbstractElasticsearchConfiguration {
@Override public RestHighLevelClient elasticsearchClient() { ClientConfiguration configuration = ClientConfiguration.builder( ) .connectedTo("192.168.100.126:9200") .build(); RestHighLevelClient client = RestClients.create(configuration).rest(); return client; } }
|
使用方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| @SpringBootTest class ElasticsearchApplicationTests {
@Autowired ElasticsearchOperations elasticsearchOperations;
@Test void testSaveByOperations(){ Book book=new Book(2,"西游记2","吴承恩二世"); IndexQuery indexQuery= new IndexQueryBuilder() .withId(book.getId().toString()) .withObject(book) .build(); String documentId = elasticsearchOperations.index(indexQuery, IndexCoordinates.of("at")); System.out.println(documentId); }
@Test void testFindByOperations(){ Book book = elasticsearchOperations.get("2",Book.class,IndexCoordinates.of("at")); System.out.println(book); }
}
|
结果
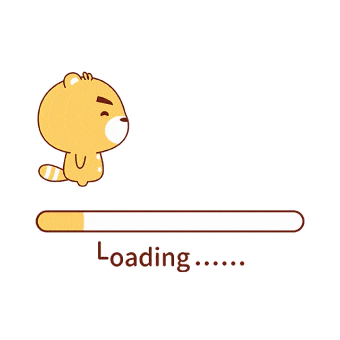
SpringBoot与检索(ElasticSearch)